Python multiprocessing Manager로 custom class 공유하기
BaseManager에 커스텀 클래스를 register하면 된다. 나머지는 그냥 원래 Manager 쓰는 거랑 똑같이 쓰면 된다.
import asyncio
import aiohttp
from multiprocessing.managers import BaseManager
class Session:
def __init__(self, beta_engine=False):
process = self._init_session()
asyncio.run(process)
async def _init_session(self):
self.session = aiohttp.ClientSession()
class MyManager(BaseManager):
pass
MyManager.register('Session', Session)
with MyManager() as manager:
session = manager.Session()
import multiprocessing
from multiprocessing.managers import BaseManager, NamespaceProxy
class Counter(object):
def __init__(self):
self.value = 0
def update(self, value):
self.value += value
def update(counter_proxy, thread_id):
counter_proxy.update(1)
class CounterManager(BaseManager):
pass
class CounterProxy(NamespaceProxy):
_exposed_ = ('__getattribute__', '__setattr__', '__delattr__', 'update')
def update(self, value):
callmethod = object.__getattribute__(self, '_callmethod')
return callmethod(self.update.__name__, (value,))
CounterManager.register('Counter', Counter, CounterProxy)
def main():
manager = CounterManager()
manager.start()
counter = manager.Counter()
pool = multiprocessing.Pool(multiprocessing.cpu_count())
for i in range(10):
pool.apply(func = update, args = (counter, i))
pool.close()
pool.join()
print 'Should be 10 but is %s.' % counter.value
if __name__ == '__main__':
main()
How to share an aiohttp.ClientSession() between processes?
I’m trying to create a shared resource in which I can use an aiohttp.ClientSession() object to fetch certain data. This allows me to use parallelization to speed up the actual calculations.However...
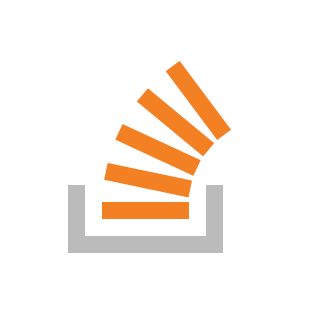
How can I share a class between processes?
I want to have global object which is shared and updated by all processes with minimum locking. import multiprocessing class Counter(object): def __init__(self): self.value = 0 def updat...
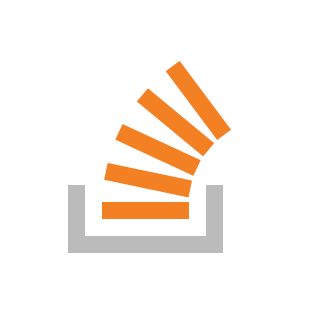
출처는 stackoverflow